Conditions in Programming Languages
What makes a programming language dynamic and useful to humankind is its ability to infer certain situations and decide the appropriate instructions to run based on the situation. Conditions are a powerful tool within programming languages that allow programmers to determine which set of instructions a program must run based on the input it receives.
For example, when you send money via an application to a friend, the application must first check if there is enough funds in your account to make the transfer. It needs to determine whether to show you a message stating you have insufficient funds or to successfully carry out the transaction and send money to your friend. If we were to create a diagram of the flow of actions involved, it would look like this:
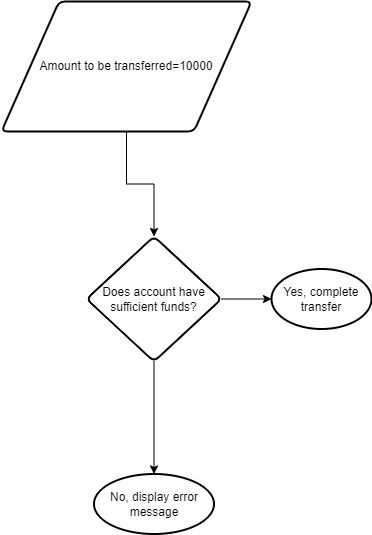
Modern programming languages allow the following decision constructs:
- If conditionals
- Switch construct
Before you read further if you are unaware of what logical operators are or do not understand data types go through these articles:
If Conditions:
if(condition){
//instructions;}
else if(condition){
//else if is an optional construct
//instructions;
}
else{
//else is an optional construct
//instructions;
}
Let's consider an example where a program is trying to understand if the user entry is a negative or a non-negative number. The program could be written in the following manner in Java using if and else statements:
//user_entry is a variable that holds the input provided by the user
if(user_entry<0){
System.out.println("The number you entered is a negative number");
}
else{ System.out.println("The number you entered is a not a negative number");
}
But what if we wanted to verify the value of an entry that could have more than one value or property. For example how would we determine if a character entered by the user is a vowel or a consonant. This logic could be charted out in the following manner:
//We assume here that the user only enters letters of the English Alphabet and no special characters
if(user_entry=='a' || user_entry=='A'){
System.out.println("Vowel detected");
}
else if(user_entry=='e' || user_entry=='E'){
System.out.println("Vowel detected");
}
else if(user_entry=='i' || user_entry=='I'){
System.out.println("Vowel detected");
}
else if(user_entry=='o' || user_entry=='O'){
System.out.println("Vowel detected");
}
else if(user_entry=='u' || user_entry=='U'){
System.out.println("Vowel detected");
}
else{
System.out.println("Consonant Detected")}
Nested if :
An if statement within another if statement is a nested if statement. If your program intends to check two conditions sequentially, you could potentially use a nested if condition. For example check if a number is a positive number and also check if the number is even.
if(num>0){
if(num%2==0) {
System.out.println("Number is a positive even number")
}
}
Note: In the above example there is no else statement. Recall that else is an optional construct. Else is mandatory only if the conditional construct involves an else if statement. 'If' statements can exist individually in any code block, where as an 'else' statement can only exist if it is preceded by an 'if' statement. Similarly 'else if' statements can only exist if they are preceded by an 'if' statement.
Compounding Conditions:
As a general practice we avoid nesting conditional statements where possible, since nesting can lead to code that is very difficult to read. You can avoid nesting by compounding sequential conditions by using logical operators. The above code can be rewritten in the following manner and it achieves the same outcome.
if(num>0 && num%2==0)
System.out.println("Number is a positive even number")
Note: If the execution block as in statements within the curly braces consists of only one statement you can skip adding braces {} around the statement.
Using the Switch Construct:
//assume user_entry is always a small case letter of the English alphabet
switch(user_entry){
case 'a': System.out.println("Vowel detected");
break;
case 'e': System.out.println("Vowel detected");
break;
case 'i': System.out.println("Vowel detected");
break;
case 'o': System.out.println("Vowel detected");
break;
case 'u': System.out.println("Vowel detected");
break;
default: System.out.println("Consonant detected");
}
The Switch construct is analogous to the if ..else if ..else construct. It is particularly useful when we are interpreting the properties of a single variable. The case statement here represents the condition with a potential value to check against. Default is an optional statement that represents a statement to execute if all cases fail or do not apply. The 'break' statement is a jump statement that tells the program to exit this construct once a case is met or holds true. For example if the user entered the letter 'e' our program would execute the statement at case 'e' and then exit the switch construct instead of checking for the remaining cases underneath it. The 'break' statement enables it to skip the remaining cases. It is important to note that the case 'a' is checked against when user_entry is 'e' since the program is always executed sequentially top-down in the order the instructions are written.